How to Read a Sentence in Java Abd Stire
How to store data in Java objects
A timely introduction to using instance variables in your Java classes

Concluding updated: January 2020
Although the snooze push is probably the near ordinarily used button on an alert clock, even a simple AlarmClock
grade needs a few more features. For instance, yous might want to control how long the alarm clock will stay in snooze mode. In order to add together such a characteristic, you need to understand how Java controls information.
Developers utilize variables in Java to concord data, with all variables having a information type and a name. The data blazon determines the values that a variable can hold. In this tutorial, you'll acquire how integral types agree whole numbers, floating point types hold existent numbers, and cord types hold graphic symbol strings. Then yous'll get started with using instance variables in your Java classes.
Variables and primitive types
Called archaic types, integral and floating signal types are the simplest information types in Java. The following program illustrates the integral blazon, which tin can hold both positive and negative whole numbers. This programme also illustrates comments, which certificate your lawmaking only don't affect the programme in any style.
/* * This is likewise a comment. The compiler ignores everything from * the outset /* until a "star slash" which ends the comment. * * Here's the "star slash" that ends the comment. */ public grade IntegerTest { public static void main(String[] args) { // Here's the declaration of an int variable called anInteger, // which you give an initial value of 100. int anInteger = 100; // Declare and initialize anInteger System.out.println(anInteger); // Outputs 100 // You lot can also practice arithmetics with primitive types, using the // standard arithmetic operators. anInteger = 100 + 100; System.out.println(anInteger); // Outputs 200 } }
Java as well uses floating indicate types, which tin hold real numbers, significant numbers that include a decimal place. Hither's an case program:
public class DoubleTest { public static void main(String[] args) { // Hither'south the declaration of a double variable called aDouble. // You also give aDouble an initial value of 5.76. double aDouble = 5.76; // Declare and initialize aDouble Organization.out.println(aDouble); // Outputs five.76 // You can also practice arithmetic with floating point types. aDouble = 5.76 + 1.45; Organisation.out.println(aDouble); // Outputs 7.21 } }
Try running the programs above. Recollect, you have to compile before you tin run them:
javac *.java java IntegerTest java DoubleTest
Java uses four integral types and two floating point types, which both concord unlike ranges of numbers and take upward varying amounts of storage space, as shown in the tables beneath.
A string blazon holds strings, and handles them differently from the style integral and floating signal types handle numbers. The Coffee linguistic communication includes a String
class to represent strings. You declare a string using the type Cord
, and initialize information technology with a quoted string, a sequence of characters contained within double quotes, equally shown below. Y'all can also combine two strings using the +
operator.
// Lawmaking fragment // Declaration of variable southward of type String, // and initialization with quoted string "Hello." Cord south = "Howdy"; // Concatenation of string in s with quoted string " Earth" Cord t = south + " Globe"; System.out.println(t); // Outputs Hello Globe
Variable scope
In addition to type, scope is also an important feature of a variable. Scope establishes when a variable is created and destroyed and where a developer tin access the variable within a program. The identify in your plan where you lot declare the variable determines its scope.
So far, I've discussed local variables, which concur temporary data that you use inside a method. You declare local variables inside methods, and you can admission them only from within those methods. This ways that yous tin retrieve merely local variables anInteger
, which y'all used in IntegerTest
, and aDouble
, which you used in DoubleTest
, from the master method in which they were declared and nowhere else.
You can declare local variables within any method. The example code below declares a local variable in the AlarmClock snooze()
method:
public form AlarmClock { public void snooze() { // Snooze time in millisecond = 5 secs long snoozeInterval = 5000; System.out.println("ZZZZZ for: " + snoozeInterval); } }
Y'all tin go to snoozeInterval
but from the snooze()
method, which is where you declared snoozeInterval, as shown here:
public class AlarmClockTest { public static void main(String[] args) { AlarmClock aClock = new AlarmClock(); aClock.snooze(); // This is still fine. // The next line of code is an ERROR. // Yous can't access snoozeInterval outside the snooze method. snoozeInterval = 10000; } }
Method parameters
A method parameter, which has a telescopic similar to a local variable, is another type of variable. Method parameters pass arguments into methods. When yous declare the method, you specify its arguments in a parameter listing. Yous pass the arguments when y'all call the method. Method parameters function similarly to local variables in that they lie inside the scope of the method to which they are linked, and tin be used throughout the method. However, unlike local variables, method parameters obtain a value from the caller when it calls a method. Here's a modification of the alert clock that allows you to pass in the snoozeInterval
.
public grade AlarmClock { public void snooze(long snoozeInterval) { Organisation.out.println("ZZZZZ for: " + snoozeInterval); } }
public class AlarmClockTest { public static void main(String[] args) { AlarmClock aClock = new AlarmClock(); // Laissez passer in the snooze interval when you call the method. aClock.snooze(10000); // Snooze for 10000 msecs. } }
Member variables: How objects store information
Local variables are useful, merely considering they provide only temporary storage, their value is express. Since their lifetimes span the length of the method in which they are declared, local variables compare to a notepad that appears every fourth dimension yous receive a phone call, but disappears when you lot hang up. That setup can exist useful for jotting down notes, simply sometimes you need something more permanent. What'due south a programmer to do? Enter member variables.
Member variables -- of which there are two, instance and static -- make upwardly part of a class.
Variable scope and lifetime
Developers implement instance variables to contain data useful to a class. An example variable differs from a local variable in the nature of its scope and its lifetime. The entire course makes up the telescopic of an example variable, not the method in which it was declared. In other words, developers tin can access instance variables anywhere in the class. In addition, the lifetime of an instance variable does not depend on any detail method of the class; that is, its lifetime is the lifetime of the instance that contains it.
Instances are the actual objects that you create from the blueprint you design in the course definition. Y'all declare instance variables in the course definition, affecting each instance y'all create from the design. Each instance contains those instance variables, and data held within the variables can vary from instance to instance.
Consider the AlarmClock
class. Passing the snoozeInterval
into the snooze()
method isn't a great design. Imagine having to type in a snooze interval on your alert clock each time you fumbled for the snooze button. Instead, simply give the whole alarm clock a snoozeInterval
. You complete this with an instance variable in the AlarmClock
class, as shown below:
public form AlarmClock { // Y'all declare snoozeInterval here. This makes it an instance variable. // You as well initialize information technology here. long m_snoozeInterval = 5000; // Snooze fourth dimension in millisecond = v secs. public void snooze() { // Yous tin still become to m_snoozeInterval in an AlarmClock method // considering you are within the scope of the grade. Organisation.out.println("ZZZZZ for: " + m_snoozeInterval); } }
You can access instance variables nearly anywhere within the class that declares them. To be technical about it, you declare the instance variable within the course scope, and you tin retrieve it from almost anywhere within that scope. Practically speaking, you can admission the variable anywhere between the kickoff curly bracket that starts the class and the endmost bracket. Since you also declare methods within the class telescopic, they besides can admission the example variables.
You lot can also access instance variables from outside the class, as long every bit an example exists, and you have a variable that references the instance. To retrieve an instance variable through an example, you utilize the dot operator together with the case. That may not be the ideal mode to admission the variable, but for at present, complete it this style for illustrative purposes:
public class AlarmClockTest { public static void chief(String[] args) { // Create two clocks. Each has its ain m_snoozeInterval AlarmClock aClock1 = new AlarmClock(); AlarmClock aClock2 = new AlarmClock(); // Modify aClock2 // Yous'll before long see that in that location are much meliorate ways to do this. aClock2.m_snoozeInterval = 10000; aClock1.snooze(); // Snooze with aClock1's interval aClock2.snooze(); // Snooze with aClock2's interval } }
Try this plan out, and you'll come across that aClock1
all the same has its interval of five,000 while aClock2
has an interval of 10,000. Once more, each instance has its own instance information.
Don't forget, the class definition is merely a blueprint, so the case variables don't actually be until you create instances from the pattern. Each instance of a class has its own copy of the instance variables, and the blueprint defines what those instance variables will be.
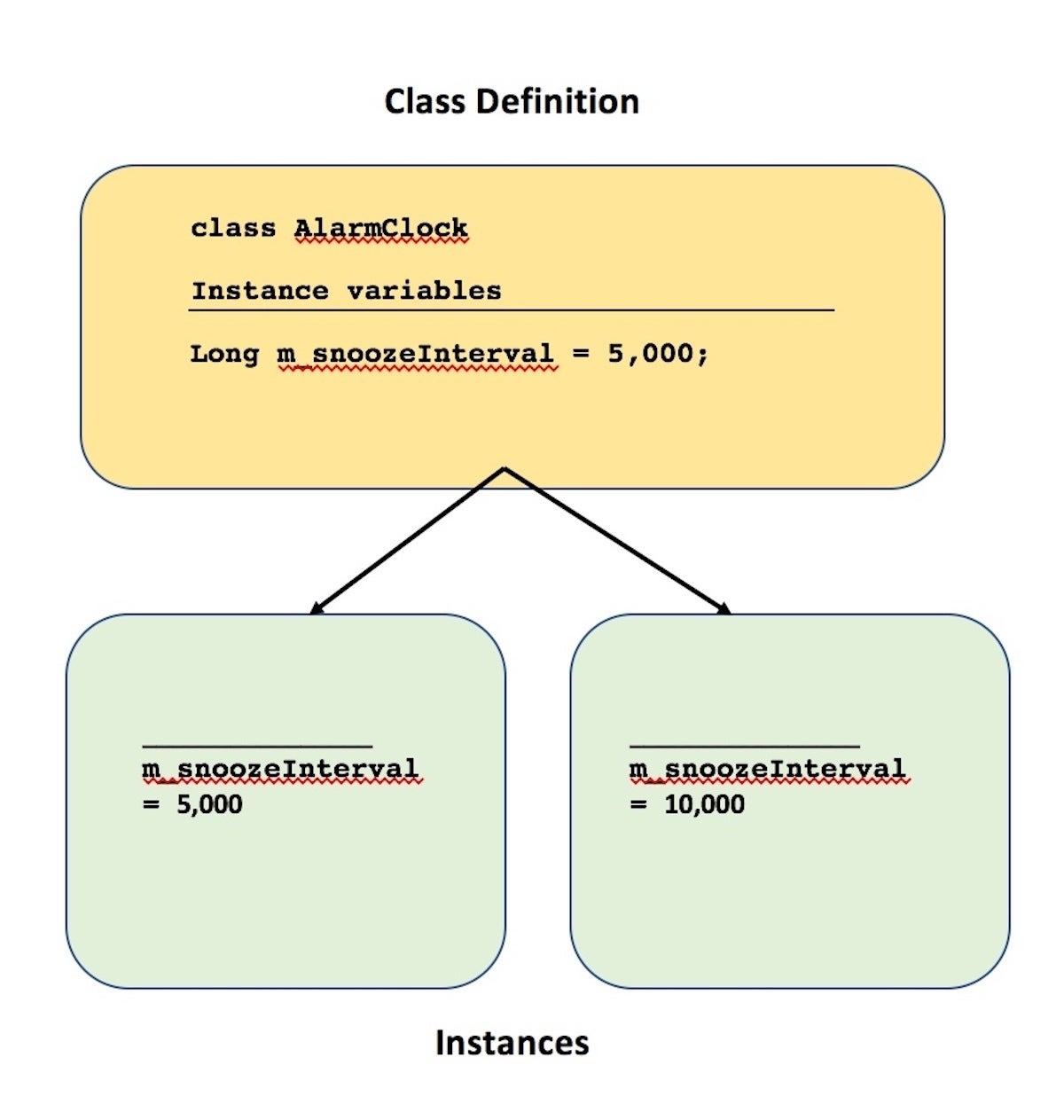
Effigy 1. Ii instances of an AlarmClock with case variables
Encapsulation
Encapsulation is i of the foundations of object-oriented programming. When using encapsulation, the user interacts with the blazon through the exposed behavior, not directly with the internal implementation. Through encapsulation, you hide the details of a type's implementation. In Java, encapsulation basically translates to this simple guideline: "Don't access your object's data directly; utilise its methods."
That is an elementary idea, but it eases our lives as programmers. Imagine, for case, that you wanted to instruct a Person
object to stand up upwardly. Without encapsulation, your commands could go something similar this: "Well, I estimate you'd need to tighten this muscle hither at the front of the leg, loosen this muscle hither at the back of the leg. Hmmm -- need to bend at the waist besides. Which muscles spark that motion? Demand to tighten these, loosen those. Whoops! Forgot the other leg. Darn. Watch it -- don't tip over ..." You get the idea. With encapsulation, you would just need to invoke the standUp()
method. Pretty easy, yes?
Some advantages to encapsulation:
- Abstraction of detail: The user interacts with a blazon at a higher level. If you use the
standUp()
method, you no longer need to know all the muscles required to initiate that motion. - Isolation from changes:Changes in internal implementation don't affect the users. If a person sprains an talocrural joint, and depends on a cane for a while, the users still invoke only the
standUp()
method. - Correctness:Users can't arbitrarily modify the insides of an object. They can simply complete what you permit them to do in the methods you write.
Hither's a brusk example in which encapsulation clearly helps in a program's accuracy:
// Bad -- doesn't utilize encapsulation public course Person { int m_age; } public class PersonTest { public static void master(String[] args) { Person p = new Person(); p.m_age = -5; // Hey -- how tin someone be minus v years one-time? } } // Amend - uses encapsulation public grade Person { int m_age; public void setAge(int age) { // Bank check to make sure historic period is greater than 0. I'll talk more well-nigh // if statements at another time. if (age > 0) { m_age = age; } } } public class PersonTest { public static void main(Cord[] args) { Person p = new Person(); p.setAge(-five); // Won't have any issue at present. } }
Even that simple program shows how you can slip into problem if you directly admission the internal data of classes. The larger and more circuitous the programme, the more important encapsulation becomes. Also remember that many programs start out small and so grow to last indefinitely, so it's essential that yous design them correctly, correct from the get-go. To apply encapsulation to AlarmClock
, y'all can just create methods to manipulate the snooze interval.
Write the program
Source: https://www.infoworld.com/article/2076301/learn-how-to-store-data-in-objects.html
0 Response to "How to Read a Sentence in Java Abd Stire"
Post a Comment